BVSEO (zip method)
Prerequisites
The following prerequisites must be satisfied before implementing SEO integration:
- You must be using the Bazaarvoice JavaScript integration method.
- You must provide an XML product feed to Bazaarvoice.
Implementing SEO
Automate the following tasks daily:
Step 1: Download SEO zip files
Every day the SEO zip files are made available on your production and staging SFTP account. Download zip files from the following locations:
feeds/default_smartseo.zip
feeds/default_smartseo.zip.ready
Step 2: Unzip the SEO files
Verify that the .ready
file exists, then download the .zip
file and extract its contents.
ready
file is created to notify you that the .zip
is completely uploaded.The following table identifies the pages that are present if any content exists.
Type of UGC | Folder | Directory |
---|---|---|
R&R | reviews |
1235-en_us/reviews/product/1/001122.htm |
Q&A | questions |
1235-en_us/questions/product/1/112233.htm
OR 1235-en_us/questions/category/1/112233.htm |
Files in these directories are named SUBJECTID.htm
, where SUBJECTID
represents the URL encoded ID of a product or category. For example, a product that features reviews and has an ID of 00+1122
, generates the following filename:
1235-en_us/reviews/product/1/00%2B1122.htm
- Products and categories without user-generated content (UGC) do not possess corresponding HTML files.
- Because the SEO snapshot is refreshed daily while JavaScript rendered content is updated throughout the day, the two versions are not always synchronized perfectly. This difference between the versions is acceptable. However, you must run the update every day to keep the versions reasonably synchronized. It's recommended that you schedule your feed pickup after 11:00 a.m. CST. This is the time that feeds are typically completed.
Step 3: Inject SEO content into product pages
After you unzip the SEO files, insert them into your product and category pages.
For each page, embed the contents of the appropriate SEO file within the main content div
element. For best results, keep the contents of each SEO file intact and unchanged as they are injected.
Ratings & Reviews
The following div
element represents the default main content for Ratings & Reviews:
<div id="BVRRContainer"></div>
The following example represents the content that must be added to this element on a product display page for a product with an ID of 001122
:
<div id="BVRRContainer">
<!-- include contents of 1235-en_us/reviews/product/1/001122.htm if it exists -->
</div>
The ID of the div
element can be customized. If you used the JavaScript API to change the ID, use the customized div
element.
Questions & Answers
The following div
element represents the default main content for Questions & Answers:
<div id="BVQAContainer"></div>
The following code represents the content that must be added to this element for a product with an ID of 001122
:
<div id="BVQAContainer">
<!-- include contents of 1235-en_us/questions/product/1/001122.htm if it exists -->
</div>
The ID of the div
element can be customized. If you used the JavaScript API to change the ID, use the customized div
element.
For a category page with an ID of 112233
, a similar change is made by using the following path:
1235-en_us/questions/category/1/112233.htm
Perform a string replacement within the SEO files
Unless pagination is disabled, Bazaarvoice generates links to all additional pages of content. However, the URLs contain a token that you must replace before they can function appropriately.
In the SEO files, the string INSERT_PAGE_URI
represents this token, which is located wherever the current page’s URL must be inserted with either a question mark (?
) or ampersand (&
) at its end.
In Ratings & Reviews, the SEO file contains a value like the following example.
<a href="{INSERT_PAGE_URI}bvrrp=1235-en_us/reviews/product/2/6789.htm">2</a>
where DisplayCode
represents your unique display code, such as 1234-en_us
. Contact
Bazaarvoice Support
to request this value from the Bazaarvoice implementation team.
If the URL of the current page is http://www.client.com/6789.htm
, the link resembles the following example after the string replacement:
<a href="http://www.client.com/6789.htm?bvrrp=1235-en_us/reviews/product/2/6789.htm">2</a>
In this example, the ?
immediately precedes the bvrrp
parameter.
If the current page’s URL already includes URL parameters, such as http://www.client.com/product.htm?id=6789
, the link resembles the following example after the string replacement:
<a href="http://www.client.com/product.htm?id=6789&bvrrp=1235-en_us/reviews/product/2/6789.htm">2</a>
In this example, the &
immediately precedes the bvrrp
parameter.
Conduct this string-replacement operation whenever you include any SEO files on your page.
Handle the SEO URL parameter
Update your product and category pages to search for a new URL parameter that Bazaarvoice passes to them. The value of this parameter indicates the path to the file that must be included in the SEO feed.
The following table identifies the default URL parameter that is associated with each Bazaarvoice product and provides an example URL for a product with an ID of 6789
.
Type of UGC | Default parameter | Example value* |
---|---|---|
Reviews | bvrrp |
{DisplayCode}/reviews/product/2/6789.htm |
In each example value, DisplayCode represents your unique display code, such as 1234-en_us. Contact
Bazaarvoice Support
to request the DisplayCode
value from the Bazaarvoice implementation team.
These parameters exist in a URL only when it links to a page other than the first one. As a result, it is recommended that you treat them as optional.
The logic that is associated with product and category pages does not always include the first page of content. As a result, you must update this logic as follows to check for the value of the SEO URL parameter:
- If the URL parameter exists and if the file to which its value refers exists in the SEO feed, include the contents of that file instead of the default first page.
- If the URL parameter does not exist or if the file to which it refers does not exist, use the default first page.
- If a default first page does not exist, do not include any content.
The following pseudocode outlines this logic for ratings and reviews.
// returns the file that should be included on the product/category
// page or null if nothing should be included
function getRRSmartSEOFile() {
var SEOParam = getURLParameter('bvrrp');
var SEOFile = '/path/to/smartseo/' + SEOParam;
var defaultSEOFile = '/path/to/smartseo/1235-en_us/reviews/product/1/' +productID + '.htm';
if ( hasValue(SEOParam) && fileExists(SEOFile) ) {
return SEOFile;
} else if ( fileExists(defaultSEOFile) ){
return defaultSEOFile;
} else {
return null;
}
}
Leverage this logic when the appropriate Bazaarvoice content area is rendered, as the following example shows.
<div id="BVRRContainer">
{ if getRRSmartSEOFile() != null then insert
stringReplaceURLs(getRRSmartSEOFile()) }
</div>
When UGC is behind a tab (special case)
If your UGC resides behind a tab on your site, place the SEO content within a div
element on your product pages. Tabbed navigation has become very common in recent years.
Search engines are capable of effectively indexing content that resides behind a tab, provided the following two conditions are met:
- The SEO content is present in the initial HTML format of the page and is not loaded through scripts on your side.
- The SEO content is visible on the page even after you turn off JavaScript in your browser and refresh the page.
"visibility: hidden"
or "display: none"
, then:
- Use JavaScript to reset this property. Do not declare this property in a stylesheet or inline to the element.
- Apply to all tabs so that all content is visible when the page is displayed with JavaScript off.
Example code
Here is an example of the JavaScript code that can be used to hide tab content:
<script type="text/javascript">
document.getElementById('tab1').style.display = 'none';
document.getElementById('tab2').style.display = 'none';
document.getElementById('tab3').style.display = 'none';
document.getElementById('tab4').style.display = 'none';
</script>
Example product page
Here is an image of a product page where the UGC is hidden (in an accordian). The consumer must click the plus sign to reveal the customer reviews.
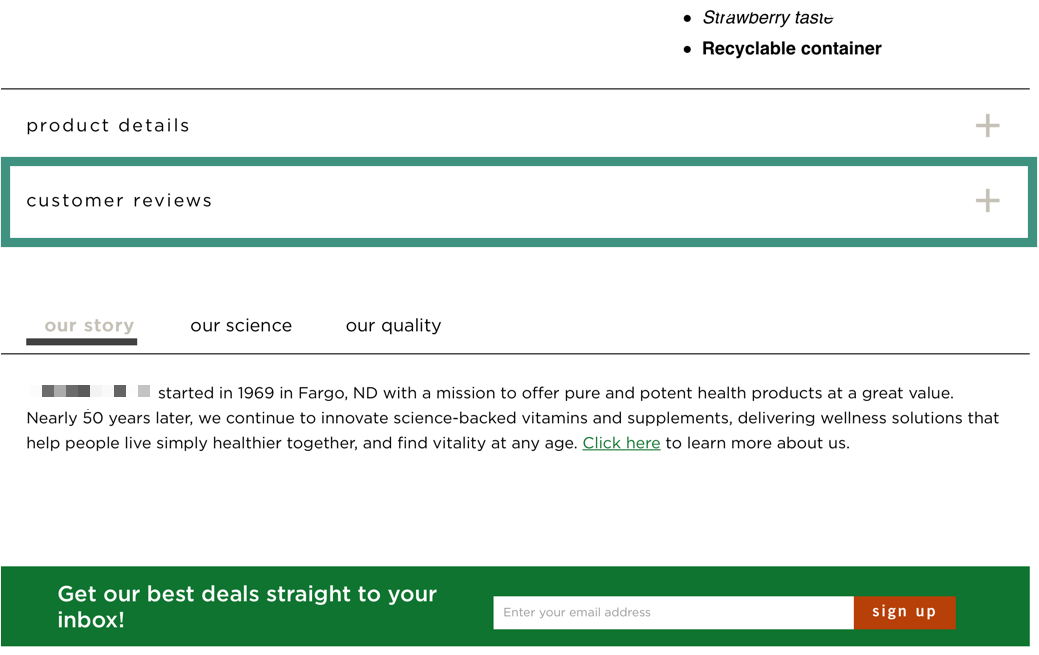