Encrypt customer PII
Customer email addresses and phone numbers are considered personally identifiable information (PII), content that could potentially distinguish or identify a specific individual. You can use encryption to ensure the security of customer email addresses and phone numbers used for notifications and requests. To encrypt the email addresses, you must add information detailed in the following sections to interaction feeds or to transactions.
Bazaarvoice email encryption is based on the RSA algorithm (4096-bit key). RSA pairs a public key to encrypt data and a private key to decrypt data. Bazaarvoice makes a shared public key available for clients, and maintains a private key.
Accessing public encryption keys
Bazaarvoice issues separate public keys for both staging and production environments, which cannot be used interchangeably.
Bazaarvoice strongly recommends that you obtain a new public key programmatically when you encrypt email addresses. Bazaarvoice changes the public and private keys regularly, and will change keys immediately if they are compromised. Older keys will continue to work, although you might receive a warning since retrieving new keys helps your company maintain the highest level of security.
You must ensure that the encryption key you use matches the correct environment. The production environment uses different keys, so PII encrypted with the staging key can’t be decrypted.
Our public key is encoded in the privacy enhanced mail (PEM) format. To avoid format issues, the encrypted data must be hex encoded.
The <EncryptedEmailAddress>
element is not added to the XML schema until version 14.5 (<Feed xmlns="https://www.bazaarvoice.com/xs/PRR/PostPurchaseFeed/14.5" >
). Clients who upgrade the feed implementation must use a 14.5 or a higher version schema (14.7 is the correct version).
You can use a single encryption key/key ID to encrypt all records in the pixel or feed. For large data sets, if you call the API for each record, the encryption key will likely update during the conversion resulting in invalid encryption.
For production, you can find the most up-to-date public key at the following URL:
For staging, you can find the most up-to-date public key at the following URL:
The JSON response at the above URLs contains the public encryption key and its associated encryption key ID. You must include the encryptionKeyId string when sending encrypted email addresses to Bazaarvoice.
The following example shows a public key and encryptionKeyId.
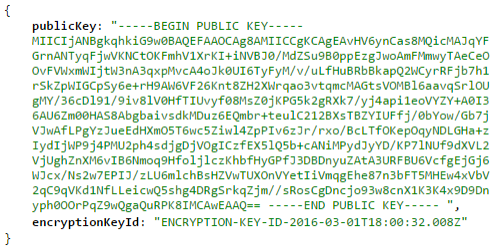
Encrypt BV Pixel PII
If you are sending interaction data automatically with BV Pixel, include the encryption key ID and encrypted email with other review request interaction parameters. Use the following parameters:
encryptedEmail
—Use this parameter instead ofemail
for customer email addresses.encryptionKeyId
—Include the encryptionKeyId string located under the public key string.
Bazaarvoice recommends the following practices when encrypting email addresses for sending interaction data automatically:
- Encrypt email addresses via a server-side operation.
- ENCRYPTION_ALGORITHM: RSA
- CIPHER_TRANSFORMATION: RSA/ECB/PKCS1Padding
- ENCRYPTION_KEY_SIZE: 4096
- Retrieve the public key programmatically at least once every day for optimal security.
- Retrieve the key no more than once each day for optimal performance.
- Store the public key within your system for error-handling resilience.
Example: Encrypted email address data in code
The following example shows the way an encrypted email address and encryption key ID would look in transaction code.
{code:javascript}
BV.pixel.trackTransaction({
"orderId" : "55555",
"tax" : "1.44",
"shipping" : "10.00",
"total" : "40.84",
"city" : "Austin",
"state" : "TX",
"country" : "USA",
"currency" : "USD",
"items" : [
{
"sku" : "2245",
"name" : "product name",
"category" : "category name",
"price" : "13.42",
"quantity" : "1",
"imageURL" : "http://test.com/1.jpg"
},
{
"sku" : "2246",
"name" : "product name2",
"imageURL" : "http://test.com/2.jpg"
}
],
"encryptedEmail" : "70c7dd458232284dd1720a29835fd4aa7afce99f2c587d3db43d19a54375b8afcdcca96206c6b3f99df8f058d1
6bf8bcb98c9b0fd52d6395d8181608e17cfabcb438c8c32415d99a5f856f041ffbb8098728c5680655dbdd68ab
c62656eb164781db3c866209b9bfeb980da66037a83b9e282a79d4e9133e21175173b8d139b64de0ad09e3b42f
9727c3a2be79682196de950179b94def664f5172ef887d31497c9d56f9196e7f8073547d95c0ccc04519102dda
ef6ff898d99c4067a1e9a5530a552f2562877618d493a2e292a181af2d8d56cb5c9dde4177da8ad1ceaf3eed12
be913bb4a7beb526f5066f82bf91048366f257754fd98b33f0aef2a5c5d63e75c7ed19059099ef56184039bae4
9d69fe554c81110a10d6bbd6244bdfa2aadcca03a6d9b6bb3fbf8979c8a98ab81969d5190da2c49b6abd1ffeaa
bded63998b0963da405b81085f7ec1b89ce9a1db6b3df88352e574881da04facb393e7b82ff8fbc78c86ed8374
389c8e160fde89f161872f5787fc39b74b3db873e498f168af726288a5b8546cfad345c8f52e9a8d3c93feae47
3df93713196d6d36dacfae7571afb6a45caa840143e433dfe52c825cf5101f050fea512edc4818c986b241e6b8
cf411daa880f7a0c3c04f94f29edc3fd89eefc3b9673ae78b6f314bc5514176965e13adf0a3b1bfb12d07e100c
e288e223443b6b82c69c5caa97d3a0d81d",
"encryptionKeyId" : "ENCRYPTION-KEY-ID-2016-07-15T21:17:55.208Z",
"locale" : "en_US"
});
{code}
Example: Email encryption using BV public key
The following is an example of email encryption using a BV public key:
/*
Example of Email Encryption using a BV Public Key
Requires:
https://www.npmjs.com/package/axios
https://www.npmjs.com/package/node-rsa
Tested Using:
"axios": "^0.21.0",
"node-rsa": "^1.1.1"
"node": "v14.4.0"
"npm": "6.14.5"
This code mirrors this bash series:
1. Extract the public key and save it to a file, converting newlines accordingly:
$ curl -s "https://stg.api.bazaarvoice.com/notifications/rsa_encryption_key/public/current/?passkey=9m5v9x6t49c4ecnwvu7av6qb" | jq -r '.publicKey' > public_staging_key.pem
$ cat public_staging_key.pem
2. Use the saved public key to encrypt a sample email address. Note that the output from OpenSSL's encryption step is binary, so we also convert the data to a hex format and remove newlines for transmission.
$ echo "jerry.garcia@email.com" | openssl rsautl -encrypt -pubin -inkey public_staging_key.pem | xxd -p | tr -d '\n' > encrypted_example_1.txt
$ cat encrypted_example_1.txt
Disclaimer: Code is provided as general guideline for integration. Developer makes no warranties, express or implied, and hereby disclaims all implied warranties, including any warranty of merchantability and warranty of fitness for a particular purpose.
*/
const axios = require('axios')
const NodeRSA = require('node-rsa');
const encodeEmail = async email => {
const res = await axios.get('https://stg.api.bazaarvoice.com/notifications/rsa_encryption_key/public/current/?passkey=9m5v9x6t49c4ecnwvu7av6qb');
const [publicKey, keyId] = [res.data.publicKey, res.data.encryptionKeyId];
//console.log(publicKey)
const key = new NodeRSA();
key.importKey(publicKey, 'pkcs8-public');
key.setOptions({encryptionScheme: 'pkcs1'});
//console.log(key.getKeySize());
const encrypted = key.encrypt(email, 'hex');
return [encrypted, keyId]
}
Encrypt interaction feed PII
If you are sending interaction data manually, add the following information to your XML feed:
<EncryptedEmailAddress>
—Use this element instead of<EmailAddress>
for customer email addresses.encryptionKeyID
—Include the encryptionKeyID string located under the public key string as an attribute of the root<Feed>
tag.
Bazaarvoice recommends the following best practices:
- For security considerations, programmatically retrieve the public key at least once every day.
- For performance considerations, retrieve the key no more than once each hour.
- For error-handling resilience, store the public key within your system.
Example: Encrypted email address data in XML feed for review requests
The following example shows the way an encrypted email address and encryption key ID would look in an interaction feed.
<Feed xmlns="https://www.bazaarvoice.com/xs/PRR/PostPurchaseFeed/14.7" encryptionKeyID="ENCRYPTION-KEY-ID-2016-07-15T21:17:55.208Z">
<Interaction>
<TransactionDate>2016-05-07T13:29:40.894</TransactionDate>
<EncryptedEmailAddress>70c7dd458232284dd1720a29835fd4aa7afce99f2c587d3db43d19a54375b8afcdcca96206c6b3f99df8f058d1
6bf8bcb98c9b0fd52d6395d8181608e17cfabcb438c8c32415d99a5f856f041ffbb8098728c5680655dbdd68abc62656eb164781db3c866209b9bfeb9
80da66037a83b9e282a79d4e9133e21175173b8d139b64de0ad09e3b42f9727c3a2be79682196de950179b94def664f5172ef887d31497c9d56f9196e
7f8073547d95c0ccc04519102ddaef6ff898d99c4067a1e9a5530a552f2562877618d493a2e292a181af2d8d56cb5c9dde4177da8ad1ceaf3eed129d6
9fe554c81110a10d6bbd6244bdfa2aadcca03a6d9b6bb3fbf8979c8a98ab81969d5190da2c49b6abd1ffeaabded63998b0963da405b81085f7ec1b89c
e9a1db6b3df88352e574881da04facb393e7b82ff8fbc78c86ed8374389c8e160fde89f161872f5787fc39b74b3db873cfad345c8f52e9a8d3c93feae
473df93713196d6d36dacfae7571afb6a45caa840143e433dfe52c825cf5101f050fea512edc4818c986b241e6b8cf411daa880f7a0c3c04f94f29edc
3fd89eefc3b9673ae78b6f314bc5514176965e13adf0a3b1bfb12d07e100ce288e223443b6b82c69c5caa97d3a0d81d</EncryptedEmailAddress>
<Locale>en_US</Locale>
<UserName>nickname1</UserName>
<UserID>718737491</UserID>
<Products>
<Product>
<ExternalId>prod01</ExternalId>
<Name>Product 1</Name>
<ImageUrl>http://test.cimages/products/01.jpg</ImageUrl>
<Price>20.61</Price>
</Product>
</Products>
</Interaction>
</Feed>
Verifying email encryption
If the email encryption is not correct, the review request import job fails to run. Use the following helpful tips to ensure a successful email encryption:
- If you encrypt the same email address more than once using the same private key, the output differs each time. You will be able to validate/match the outputs by decrypting the encrypted strings using the same private key used to encrypt.
- If you encrypt the email addresses by any method other than the recommended one, using that input will result in errors in the tool.